Understanding Functions
A Subroutine or what we will just refer to as a function is a block of code written to perform a specific task. We have actually been using functions all along our programming journey so far and you probably have not even noticed. Many functions are built into most programming languages, like the print() function for example. In python we usually place our code in main(), which is a function. In C, C++ or Java the compiler looks for the main() function to start running the program. You can also create functions to do specific calculations, like converting temperature from Celsius to Fahrenheit for example. This type of conversion is very common and we might want to use it in another program (re-usability is very important in programming; why re-invent the wheel, just use someone else’s code, as long as you can trust that it will work!).
Functions need to have two (2) seperate mechanisms to work correctly. You need a way to create the function in your program and you also need a way to then “call” the function and get the code inside of it to run. Although not normally needed for the computer, we usually place the function definition before we call the function, so that when people are actually reading the code they have seen the function definition and know what it will do before they see it being called.
This is a good template for keeping all the parts of your program organized in a program file:
comment header
global (to the file) constant(s)
global (to the file) variable(s)
function(s)
main body of code
Creating and Calling a Function
Each programming language has its own syntax to create and call a function. Here is an example program that uses functions to calculate area and perimeter (I know there are many ways to improve this code, we will be doing that in the next sections)
Code for Creating and Calling a Function
1// Copyright (c) 2020 Mr. Coxall All rights reserved.
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program uses user defined functions
6
7#include <stdio.h>
8
9void calculateArea()
10{
11 // input
12 printf("Enter the length of a rectangle (cm): ");
13 int length;
14 scanf("%d", &length);
15 printf("Enter the width of a rectangle (cm): ");
16 int width;
17 scanf("%d", &width);
18
19 // process
20 int area = length * width;
21
22 // output
23 printf("The area is %d cm²\n\n", area);
24}
25
26void calculatePerimeter()
27{
28 // input
29 printf("Enter the length of a rectangle (cm): ");
30 int length;
31 scanf("%d", &length);
32 printf("Enter the width of a rectangle (cm): ");
33 int width;
34 scanf("%d", &width);
35
36 // process
37 int perimeter = 2 * (length + width);
38
39 // output
40 printf("The perimeter is %d cm\n\n", perimeter);
41}
42
43int main() {
44 // call functions
45 calculateArea();
46 calculatePerimeter();
47
48 printf("\nDone.\n");
49 return 0;
50}
1// Copyright (c) 2020 Mr. Coxall All rights reserved.
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program uses user defined functions
6
7#include <iostream>
8
9void calculateArea() {
10 // input
11 std::cout << "Enter the length of a rectangle (cm): ";
12 int length;
13 std::cin >> length;
14 std::cout << "Enter the width of a rectangle (cm): ";
15 int width;
16 std::cin >> width;
17
18 // process
19 int area = length * width;
20
21 // output
22 std::cout << "The area is " << area << " cm²\n\n";
23}
24
25void calculatePerimeter() {
26 // input
27 std::cout << "Enter the length of a rectangle (cm): ";
28 int length;
29 std::cin >> length;
30 std::cout << "Enter the width of a rectangle (cm): ";
31 int width;
32 std::cin >> width;
33
34 // process
35 int perimeter = 2 * (length + width);
36
37 // output
38 std::cout << "The perimeter is " << perimeter << " cm\n\n";
39}
40
41int main() {
42 // call functions
43 calculateArea();
44 calculatePerimeter();
45
46 std::cout << "Done." << std::endl;
47 return 0;
48}
1/* Created by: Mr. Coxall
2 * Created on: Sep 2020
3 * This program uses user defined functions
4*/
5
6using System;
7
8/*
9 * The Program class
10 * Contains all methods for performing basic variable usage
11*/
12class Program {
13 static void calculateArea() {
14 // input
15 Console.Write("Enter the length of a rectangle (cm): ");
16 int length = int.Parse(Console.ReadLine());
17 Console.Write("Enter the width of a rectangle (cm): ");
18 int width = int.Parse(Console.ReadLine());
19
20 // process
21 int area = length * width;
22
23 // output
24 Console.WriteLine($"The area is {area} cm²\n");
25 }
26
27 static void calculatePerimeter() {
28 // input
29 Console.Write("Enter the length of a rectangle (cm): ");
30 int length = int.Parse(Console.ReadLine());
31 Console.Write("Enter the width of a rectangle (cm): ");
32 int width = int.Parse(Console.ReadLine());
33
34 // process
35 int perimeter = 2 * (length + width);
36
37 // output
38 Console.WriteLine($"The perimeter is {perimeter} cm\n");
39 }
40
41 public static void Main (string[] args) {
42 // call functions
43 calculateArea();
44 calculatePerimeter();
45
46 Console.WriteLine("\nDone.");
47 }
48}
1/**
2 * Created by: Mr. Coxall
3 * Created on: Sep 2020
4 * This program uses user defined functions
5 */
6
7package main
8
9import (
10 "fmt"
11)
12
13func calculateArea() {
14 // input
15 var length, width int
16 fmt.Print("Enter the length of a rectangle (cm): ")
17 fmt.Scanln(&length)
18 fmt.Print("Enter the width of a rectangle (cm): ")
19 fmt.Scanln(&width)
20
21 // process
22 area := length * width
23
24 // output
25 fmt.Printf("The area is %d cm²\n\n", area)
26}
27
28func calculatePerimeter() {
29 // input
30 var length, width int
31 fmt.Print("Enter the length of a rectangle (cm): ")
32 fmt.Scanln(&length)
33 fmt.Print("Enter the width of a rectangle (cm): ")
34 fmt.Scanln(&width)
35
36 // process
37 perimeter := 2 * (length + width)
38
39 // output
40 fmt.Printf("The perimeter is %d cm\n\n", perimeter)
41}
42
43func main() {
44 // call functions
45 calculateArea()
46 calculatePerimeter()
47
48 fmt.Println("\nDone.")
49}
1/*
2 * This program uses user defined functions
3 *
4 * @author Mr Coxall
5 * @version 1.0
6 * @since 2020-09-01
7 */
8
9import java.util.Scanner;
10
11final class Main {
12 /**
13 * Calculates area of rectangle.
14 *
15 * @param args nothing passed in
16 */
17 public static void calculateArea() {
18 // input
19 Scanner scanner = new Scanner(System.in);
20 System.out.print("Enter the length of a rectangle (cm): ");
21 int length = scanner.nextInt();
22 System.out.print("Enter the width of a rectangle (cm): ");
23 int width = scanner.nextInt();
24
25 // process
26 int area = length * width;
27
28 // output
29 System.out.printf("The area is %d cm²%n%n", area);
30 }
31
32 /**
33 * Calculates perimeter of rectangle.
34 *
35 * @param args nothing passed in
36 */
37 public static void calculatePerimeter() {
38 // input
39 Scanner scanner = new Scanner(System.in);
40 System.out.print("Enter the length of a rectangle (cm): ");
41 int length = scanner.nextInt();
42 System.out.print("Enter the width of a rectangle (cm): ");
43 int width = scanner.nextInt();
44
45 // process
46 int perimeter = 2 * (length + width);
47
48 // output
49 System.out.printf("The perimeter is %d cm%n%n", perimeter);
50 }
51
52 private Main() {
53 // Prevent instantiation
54 // Optional: throw an exception e.g. AssertionError
55 // if this ever *is* called
56 throw new IllegalStateException("Cannot be instantiated");
57 }
58
59 /**
60 * Main entry point into program.
61 *
62 * @param args nothing passed in
63 */
64 public static void main(final String[] args) {
65 // call functions
66 calculateArea();
67 calculatePerimeter();
68
69 System.out.println("\nDone.");
70 }
71}
1/**
2 * Created by: Mr. Coxall
3 * Created on: Sep 2020
4 * This program uses user defined functions
5 */
6
7const prompt = require('prompt-sync')();
8
9function calculateArea() {
10 // input
11 const length = parseInt(prompt("Enter the length of a rectangle (cm): "));
12 const width = parseInt(prompt("Enter the width of a rectangle (cm): "));
13
14 // process
15 const area = length * width;
16
17 // output
18 console.log(`The area is ${area} cm²\n`);
19}
20
21function calculatePerimeter() {
22 // input
23 const length = parseInt(prompt("Enter the length of a rectangle (cm): "));
24 const width = parseInt(prompt("Enter the width of a rectangle (cm): "));
25
26 // process
27 const perimeter = 2 * (length + width);
28
29 // output
30 console.log(`The perimeter is ${perimeter} cm\n`);
31}
32
33// call functions
34calculateArea();
35calculatePerimeter();
36
37console.log("Done.");
1#!/usr/bin/env python3
2"""
3Created by: Mr. Coxall
4Created on: Sep 2020
5This module uses user defined functions
6"""
7
8
9def calculate_area() -> None:
10 """The calculate_area() function calculates area of a rectangle, returns None."""
11
12 # input
13 length = int(input("Enter the length of a rectangle (cm): "))
14 width = int(input("Enter the width of a rectangle (cm): "))
15
16 # process
17 area = length * width
18
19 # output
20 print(f"The area is {area} cm²")
21 print("")
22
23
24def calculate_perimeter() -> None:
25 """The calculate_perimeter() function calculates perimeter of a rectangle, returns None."""
26
27 # input
28 length = int(input("Enter the length of a rectangle (cm): "))
29 width = int(input("Enter the width of a rectangle (cm): "))
30
31 # process
32 perimeter = 2 * (length + width)
33
34 # output
35 print(f"The perimeter is {perimeter} cm")
36 print("")
37
38
39def main() -> None:
40 """The main() function just calls other functions, returns None."""
41
42 # call functions
43 calculate_area()
44 calculate_perimeter()
45
46 print("\nDone.")
47
48
49if __name__ == "__main__":
50 main()
Example Output
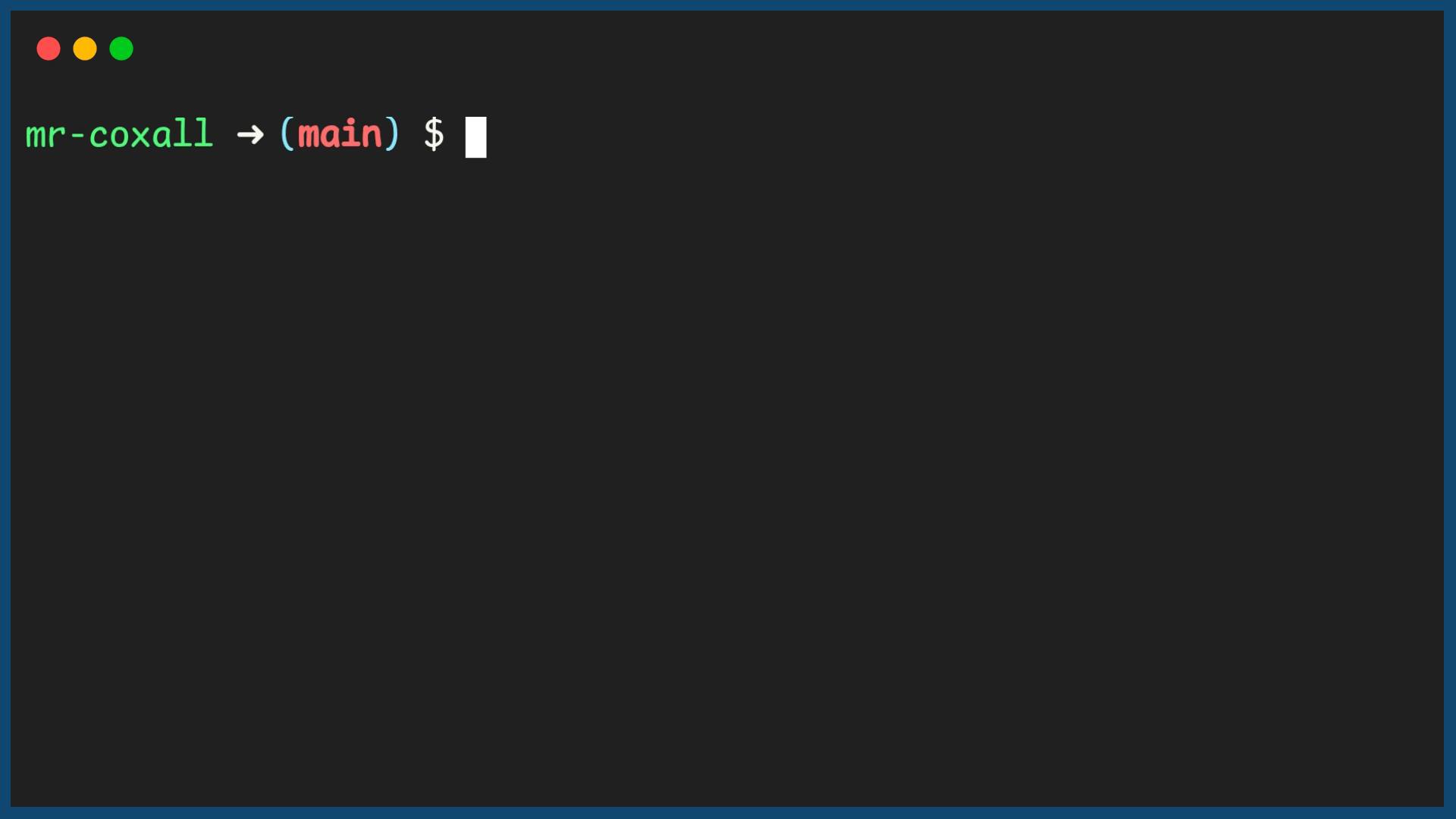