Default Values
All of the functions that we have looked at to this point, you had to ensure that you were sending the exact same number of parameters to the function as it was expecting. To help us do this a good IDE will have, “auto-complete” giving us a little pop out window to show us what should be passed over to the function.
Some built in functions we have been using can be accessed in multiple different ways though. For example in Python there is a built in function called random.randrange(). It is kind of like random.ranint() that we have used in the past. Here is the definition for random.ranint():
random.randint(a, b)
// Return a random integer N such that a <= N <= b. Alias for randrange(a, b+1).
Notice that “a & b” are our starting and ending points.
Here is the definition for random.randrange():
random.randrange(start, stop[, step])
// The positional argument pattern matches that of range().
First off there is actually 2 seperate ways we could call this function:
random.randrange(start, stop)
random.randrange(start, stop, step)
It seems that step is, “optional” which it is. By default, if you do not provide it, then python assumes the value is just 1. You can choose for example to place in a 2, and then only even numbers will be chosen. Here is how we would define the function random.randrange() to get this optional parameter:
def randrange(start, stop, step = 1):
Notice that right in the declaration of the function the, “default optional parameter” is being set. If it is not provided as a parameter, the default value is just used. Each programming language has its own syntax to make this kind of optional parameter work. Here is an example:
Code for Function with Default Values
1// No default values for functions in C
1// Copyright (c) 2020 St. Mother Teresa HS All rights reserved.
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program prints out your name, using default function parameters
6
7#include <iostream>
8
9std::string FullName(std::string firstName, std::string lastName,
10 std::string middleName = "") {
11 // return the full formal name
12
13 std::string fullName;
14
15 fullName = firstName;
16 if (middleName.size() != 0) {
17 fullName = fullName + " " + middleName[0] + ".";
18 }
19 fullName = fullName + " " + lastName;
20
21 return fullName;
22}
23
24
25int main() {
26 // gets a users name and prints out their formal name
27
28 std::string firstName;
29 std::string question;
30 std::string middleName = "";
31 std::string lastName;
32 std::string fullName;
33
34 // input
35 std::cout << "Enter your first name: ";
36 std::cin >> firstName;
37 std::cout << "Do you have a middle name? (y/n): ";
38 std::cin >> question;
39 if (question == "Y" || question == "YES" || question == "y") {
40 std::cout << "Enter your middle name: ";
41 std::cin >> middleName;
42 }
43 std::cout << "Enter your last name: ";
44 std::cin >> lastName;
45 std::cout << std::endl;
46
47 // call functions
48 if (middleName != "") {
49 fullName = FullName(firstName, lastName, middleName);
50 } else {
51 fullName = FullName(firstName, lastName);
52 }
53 std::cout << "Your formal name is " << fullName << "." << std::endl;
54
55 std::cout << "\nDone." << std::endl;
56 return 0;
57}
1/* Created by: Mr. Coxall
2 * Created on: Sep 2020
3 * This program prints out your name, using default function parameters
4*/
5
6using System;
7
8/*
9 * The Program class
10 * Contains all methods for performing how local and global variables work
11*/
12class Program {
13
14 static string FullName(string firstName, string lastName, string middleName = "") {
15 // this function calculates the full name
16 string fullName;
17
18 if (middleName == "") {
19 fullName = firstName + " " + lastName;
20 } else {
21 fullName = firstName + " " + middleName + " " + lastName;
22 }
23 return fullName;
24 }
25
26 public static void Main (string[] args) {
27 // this function gets a users name and prints out their formal name
28 string firstName;
29 string question;
30 string middleName = "";
31 string lastName;
32 string fullName;
33
34 //input
35 Console.Write("Enter your first name: ");
36 firstName = Console.ReadLine();
37 Console.Write("Do you have a middle name? (y/n): ");
38 question = Console.ReadLine();
39 if (question.ToUpper() == "Y" || question.ToUpper() == "YES") {
40 Console.Write("Enter your middle name: ");
41 middleName = Console.ReadLine();
42 }
43 Console.Write("Enter your last name: ");
44 lastName = Console.ReadLine();
45 Console.WriteLine();
46
47 // process & output
48 if (middleName != "") {
49 fullName = FullName(firstName, lastName, middleName);
50 } else {
51 fullName = FullName(firstName, lastName);
52 }
53 Console.WriteLine($"Your formal name is {fullName}.");
54
55 Console.WriteLine ("\nDone.");
56 }
57}
1// No default values for functions in Go
1/*
2 * This program shows how local and global variables work
3 *
4 * @author Mr Coxall
5 * @version 1.0
6 * @since 2020-09-01
7 */
8
9import java.util.Scanner; // Import the Scanner class
10
11final class Main {
12 private Main() {
13 // Prevent instantiation
14 // Optional: throw an exception e.g. AssertionError
15 // if this ever *is* called
16 throw new IllegalStateException("Cannot be instantiated");
17 }
18
19 /**
20 * Main entry point into program.
21 *
22 * @param args nothing passed in
23 */
24 public static void main(final String[] args) {
25 // this function calculates total from subtotal and tax
26 final float HST = 0.13f;
27 float tax;
28 float subTotal;
29 float total;
30
31 Scanner scanner = new Scanner(System.in);
32
33 // input
34 System.out.print("Enter the subtotal: $");
35 subTotal = scanner.nextFloat();
36
37 // process
38 tax = subTotal * HST;
39 total = subTotal + tax;
40
41 // output
42 System.out.println();
43 System.out.printf("The HST is: $%.2f.\n", tax);
44 System.out.printf("The total cost is: $%.2f.\n", total);
45
46 System.out.println("\nDone.");
47 }
48}
1// Copyright (c) 2020 Mr. Coxall All rights reserved
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program prints out your name, using default function parameters
6
7const prompt = require('prompt-sync')()
8
9function FullName(firstName, lastName, middleName = "") {
10 // return the full formal name
11 let fullName = firstName
12
13 if (middleName.length != 0) {
14 fullName = fullName + " " + middleName[0] + "."
15 }
16 fullName = fullName + " " + lastName
17
18 return fullName
19}
20
21// this function function calculates the full name
22let middleName = ""
23
24// input
25let firstName = prompt('Enter your first name: ')
26let question = prompt('Do you have a middle name? (y/n): ')
27
28if (question.toUpperCase() == "Y" || question.toUpperCase() == "YES") {
29 middleName = prompt('Enter your middle name: ')
30}
31let lastName = prompt('Enter your last name: ')
32
33// call functions
34let fullName = ""
35
36if (middleName != "") {
37 fullName = FullName(firstName, lastName, middleName)
38} else {
39 fullName = FullName(firstName, lastName)
40}
41console.log(`\nYour formal name is ${fullName}.`)
42
43console.log("\nDone.")
1#!/usr/bin/env python3
2"""
3Created by: Mr. Coxall
4Created on: Sep 2020
5This module prints out your name, using default function parameters
6"""
7
8
9def full_name(first_name: str, last_name: str, middle_name: str | None = None) -> str:
10 """The full_name() function calculates the full formal name, returns str."""
11 # return the full formal name
12
13 full_name = first_name
14 if middle_name is not None:
15 full_name = full_name + " " + middle_name[0] + "."
16 full_name = full_name + " " + last_name
17
18 return full_name
19
20
21def main() -> None:
22 """The main() function gets a users name and prints out their formal name, returns None."""
23
24 middle_name = None
25
26 # input & process
27 first_name = input("Enter your first name: ")
28 question = input("Do you have a middle name? (y/n): ")
29 if question.upper() == "Y" or question.upper() == "YES":
30 middle_name = input("Enter your middle name: ")
31 last_name = input("Enter your last name: ")
32
33 if middle_name is not None:
34 name = full_name(first_name, last_name, middle_name)
35 else:
36 name = full_name(first_name, last_name)
37
38 # output
39 print(f"\nYour formal name is {name}.")
40
41 print("\nDone.")
42
43
44if __name__ == "__main__":
45 main()
Example Output
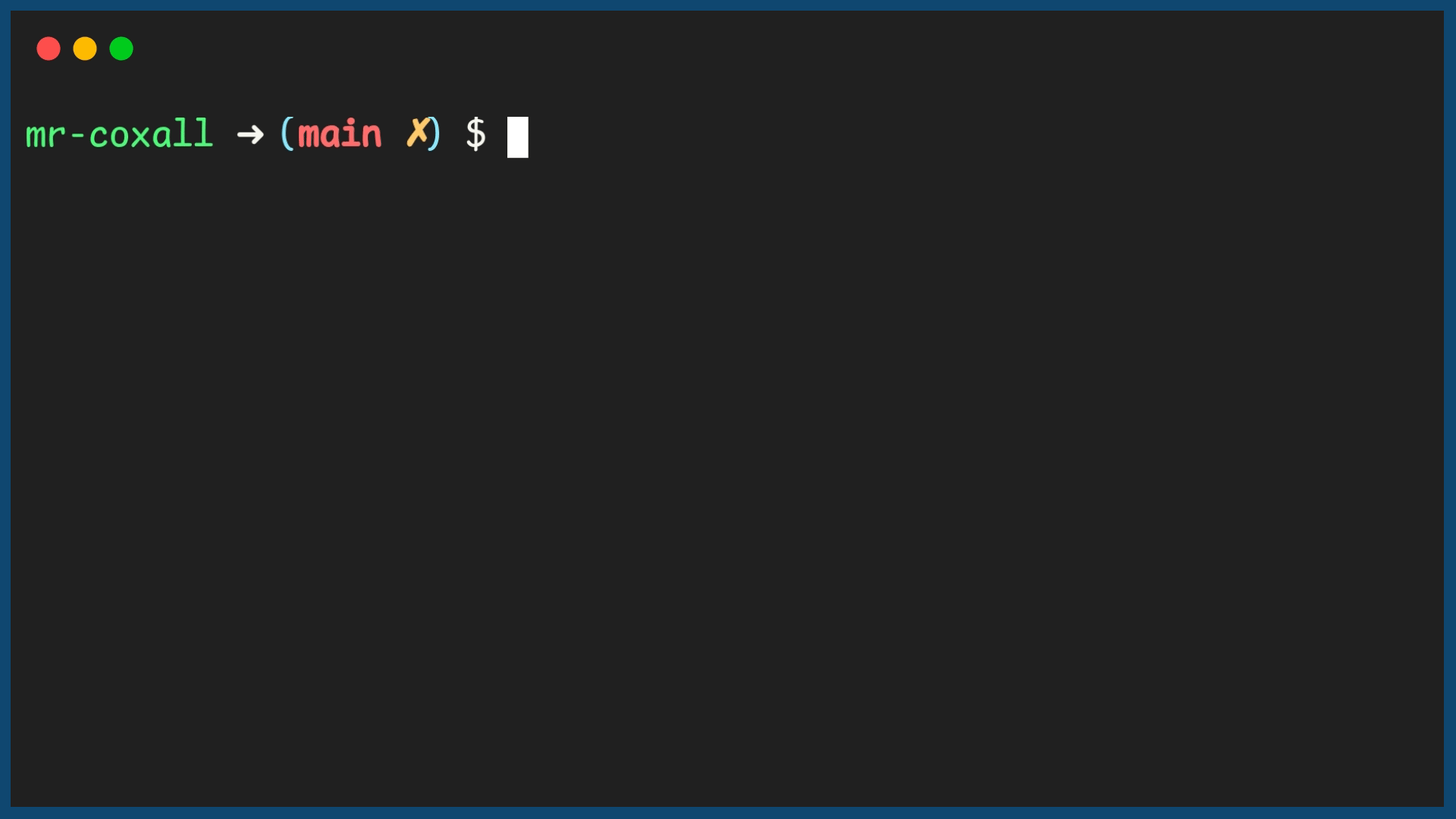