Return Values
If this was math class, the definition of a mathimatical function might be, “… a function is a relation between sets that associates to every element of a first set exactly one element of the second set,” which is a fancy way to say that if you put in a particular number into the function you get one and only one number out and it will always be the same value. The key is that you actually get something back. In computer science it is also common for functions to give you something back and we normally call this a return value or statement.
We have already seen many built in functions that have a return value, like the square root function:
Code for Function with a Return Value
// square root function
someVariable = sqrt(someNumber);
// square root function
someVariable = sqrt(someNumber);
// square root function
someVariable = sqrt(someNumber);
// square root function
someVariable = math.Sqrt(someNumber);
// square root function
someVariable = sqrt(someNumber);
// square root function
someVariable = sqrt(someNumber);
# square root function
some_variable = math.sqrt(some_number)
You will notice that the function is now on the right hand side of an assignment statement and the calculated value is being placed right into another variable. To allow this ability, we normally use the reserved word “return” to pass the value back. In many programming languages, in the definition of the function you must specify what type of variable will be returned. This way the IDE can confirm that the same types are being passed back and placed into a variable of the same type. This way the language remains type safe.
Now that we know how to use a return statement, we should no longer print out results inside a function like in the last few chapters. It is much better style to retrun the value from a function and let the calling process decide what to do with it. Here is the example from last section, this time using return values:
Example Output
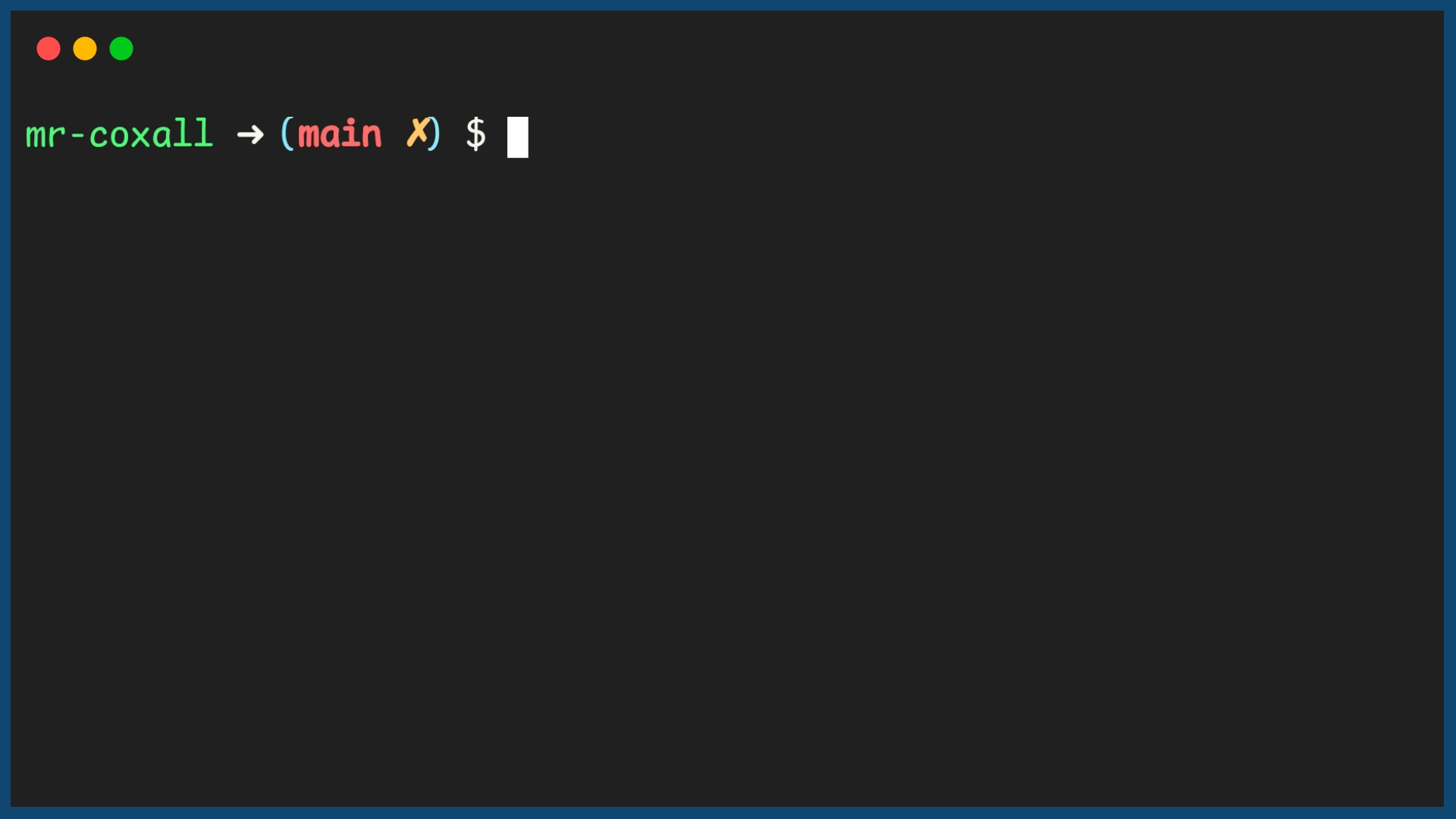