Nested Loops
The placing of one loop inside the body of another loop is called nesting. When you, “nest” two loops, the outer loop takes control of the number of complete repetitions of the inner loop. How this works is that the first pass of the outer loop triggers the inner loop, which executes to completion. Then the second pass of the outer loop triggers the inner loop again. This repeats until the outer loop finishes.
A Nested for loop (in most computer programming languages), takes the generic form of:
FOR counter1 in range(n)
FOR counter2 in range(m)
statement(s)
…
END
END
or using While loops:
WHILE counter1 <= n :
WHILE counter2 <= m :
statement(s)
…
counter2 = counter2 + 1
END
counter2 = 0
…
counter1 = counter1 + 1
END
In this example program, the output shows a 2 digit odometer, using a Nested loop.
Top-Down Design for Nested loops
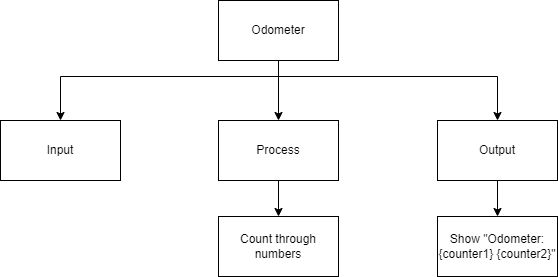
Flowchart for Nested loops
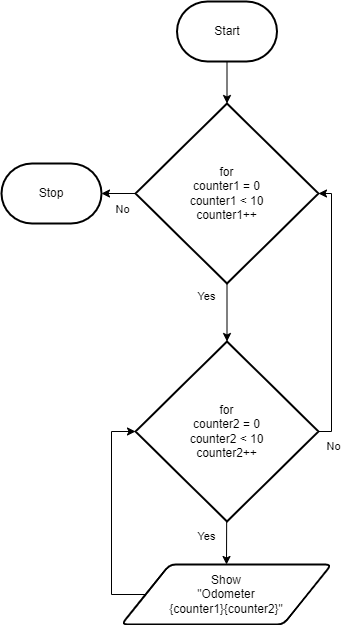
Pseudocode for Nested loops
FOR counter1 in range(10)
FOR counter2 in range(10)
SHOW Odometer {counter1}{counter2}
END
END
Code for the Nested loops
Example Output
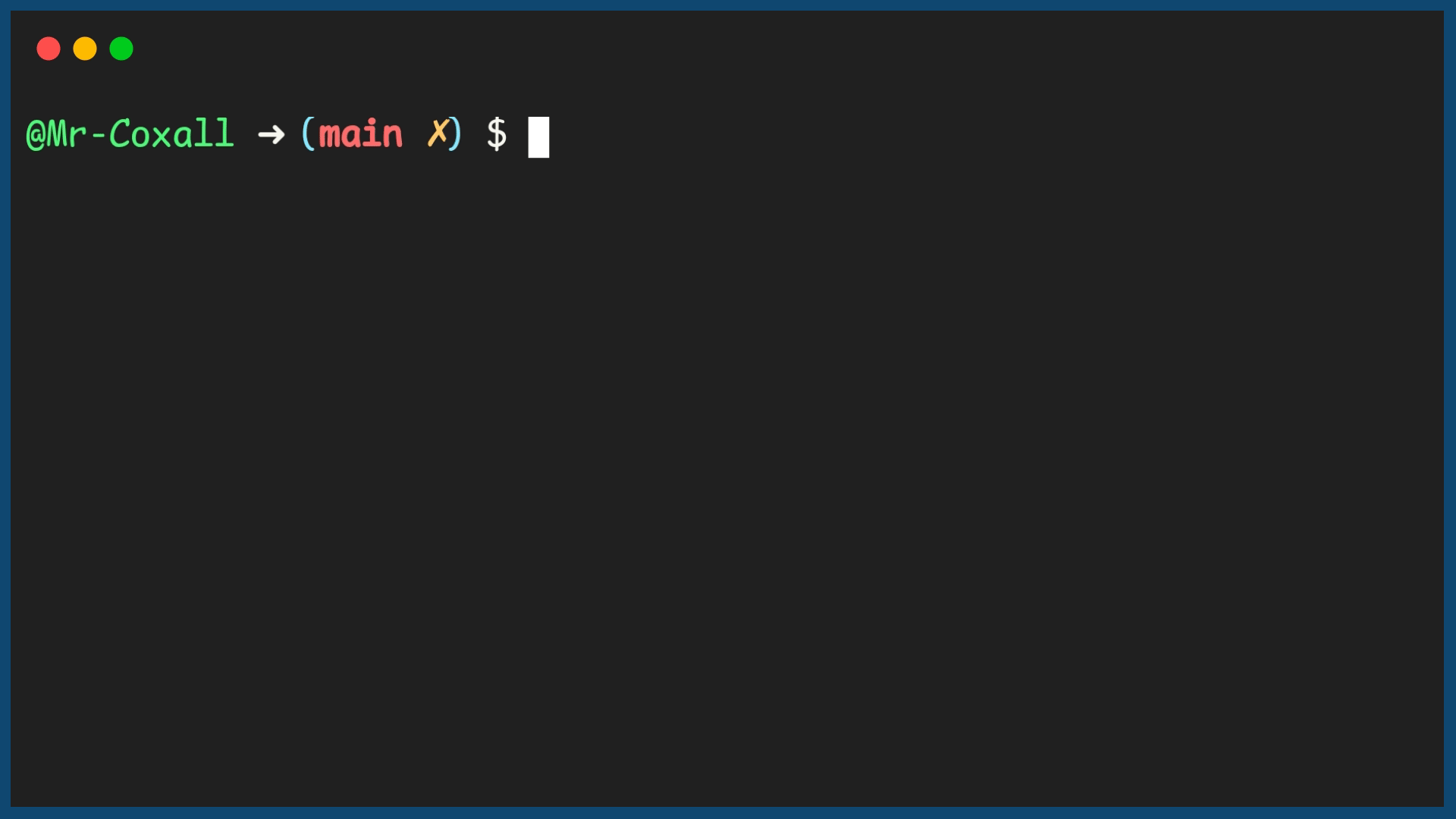