Continue Statement
The Continue statement gives you the option to skip over the part of a loop where an external condition is triggered, but to go on to complete the rest of the loop iterations. That is, the current iteration of the loop will be disrupted, but the program will return to the top of the loop. The Continue statement will be within the block of code under the Loop statement, usually after a conditional if statement.
The continue statement (in most computer programming languages), takes the generic form of:
WHILE Boolean expression
statement_1
…
IF Boolean expression THEN
CONTINUE
ENDIF
statement_2
…
counter = counter + 1
ENDWHILE
FOR counter in range(n)
statement_1
…
IF Boolean expression THEN
CONTINUE
ENDIF
statement_2
…
ENDFOR
In this example program, the user is asked to enter a positive integer and the program will count how many times it goes through the loop until it reaches that number, except it will always skip 5!
Top-Down Design for the Continue Statement
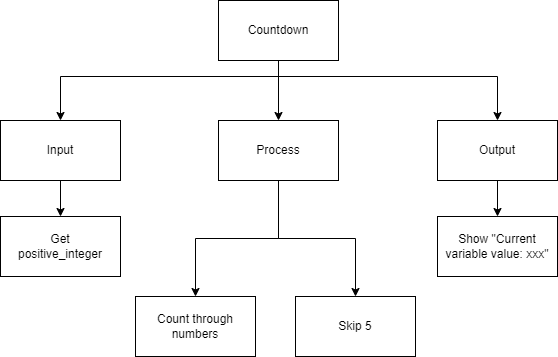
Flowchart for the Continue Statement
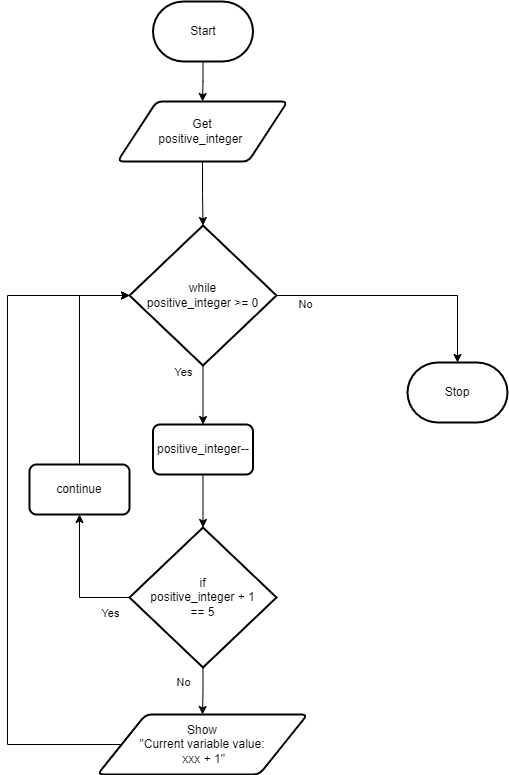
Pseudocode for the Continue Statement
GET positive_integer
WHILE (counter < positive_integer)
counter = counter - 1
IF (counter + 1 == 5) THEN
CONTINUE
ENDIF
SHOW counter + 1
ENDFOR
Code for the Continue Statement
1// Copyright (c) 2020 Mr. Coxall All rights reserved.
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program uses a for loop
6
7#include <stdio.h>
8
9int main() {
10 // this function uses a for loop
11 int positiveInteger;
12
13 // input
14 printf("Enter how many times to repeat: ");
15 scanf("%d", &positiveInteger);
16 printf("\n");
17
18 // process & output
19 while (positiveInteger >= 0) {
20 // yes, this is the exception on placing the counter at the top
21 // if you did not, then there would be an infinit loop
22
23 positiveInteger--;
24 if (positiveInteger + 1 == 5) {
25 continue;
26 }
27 printf("Current variable value: %d\n", positiveInteger + 1);
28 }
29
30 printf("\nDone.\n");
31 return 0;
32}
1// Copyright (c) 2020 St. Mother Teresa HS All rights reserved.
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program uses a for loop
6
7#include <iostream>
8
9int main() {
10 // this function uses a for loop
11 int positiveInteger;
12
13 // input
14 std::cout << "Enter how many times to repeat: ";
15 std::cin >> positiveInteger;
16 std::cout << std::endl;
17
18 // process & output
19 while (positiveInteger >= 0) {
20 // yes, this is the exception on placing the counter at the top
21 // if you did not, then there would be an infinit loop
22
23 positiveInteger--;
24 if (positiveInteger + 1 == 5) {
25 continue;
26 }
27 std::cout << "Current variable value: " <<
28 positiveInteger + 1 << std::endl;
29 }
30
31 std::cout << "\nDone." << std::endl;
32 return 0;
33}
1/* Created by: Mr. Coxall
2 * Created on: Sep 2020
3 * This program uses a for loop
4*/
5
6using System;
7
8/*
9 * The Program class
10*/
11class Program {
12 static void Main() {
13 // this function uses a do ... while loop
14
15 int positiveInteger;
16
17 // input
18 Console.Write("Enter how many times to repeat: ");
19 positiveInteger = Convert.ToInt32(Console.ReadLine());
20 Console.WriteLine();
21
22 // process & output
23 while (positiveInteger >= 0) {
24 // yes, this is the exception on placing the counter at the top
25 // if you did not, then there would be an infinit loop
26
27 positiveInteger--;
28 if (positiveInteger + 1 == 5) {
29 continue;
30 }
31 Console.WriteLine("Current variable value: " + (positiveInteger + 1));
32 }
33
34 Console.WriteLine("\nDone.");
35 }
36}
1/**
2 * Created by: Mr. Coxall
3 * Created on: Sep 2020
4 * This program uses a continue statement
5 */
6
7package main
8
9import (
10 "fmt"
11)
12
13func main() {
14 // this function uses a continue statement
15
16 var positiveInteger int
17
18 // input
19 fmt.Print("Enter a count-down number (ex: 10): ")
20 fmt.Scan(&positiveInteger)
21 fmt.Println()
22
23 // process & output
24 for positiveInteger >= 0 {
25 // yes, this is the exception on placing the counter at the top
26 // if you did not, then there would be an infinite loop
27
28 positiveInteger--
29
30 if positiveInteger+1 == 5 {
31 continue
32 }
33 fmt.Printf("Current variable value: %d\n", positiveInteger+1)
34 }
35
36 fmt.Println("\nDone.")
37}
1/*
2 * This program uses a continue statement
3 *
4 * @author Mr Coxall
5 * @version 1.0
6 * @since 2020-09-01
7 */
8
9import java.util.Scanner;
10
11public class Main {
12 public static void main(String[] args) {
13 // this function uses a continue statement
14
15 // create Scanner object for user input
16 Scanner scanner = new Scanner(System.in);
17
18 // input
19 System.out.print("Enter a count-down number (ex: 10): ");
20 String positiveIntegerStr = scanner.nextLine();
21 System.out.println();
22
23 // process & output
24 int positiveInteger = Integer.parseInt(positiveIntegerStr);
25
26 while (positiveInteger >= 0) {
27 // yes, this is the exception on placing the counter at the top
28 // if you did not, then there would be an infinit loop
29
30 positiveInteger--;
31 if (positiveInteger + 1 == 5) {
32 continue;
33 }
34 System.out.println("Current variable value: %d".formatted(positiveInteger + 1));
35 }
36
37 // close the Scanner object
38 scanner.close();
39 System.out.println("\nDone.");
40 }
41}
1/* Created by: Mr. Coxall
2 * Created on: Sep 2020
3 * This program uses a continue statement
4 */
5
6const prompt = require("prompt-sync")()
7
8let counter = 0
9
10// input
11const positiveIntegerStr = prompt("Enter a count-down number (ex: 10): ")
12console.log("")
13
14// process & output
15let positiveInteger = parseInt(positiveIntegerStr)
16
17while (positiveInteger >= 0) {
18 // yes, this is the exception on placing the counter at the top
19 // if you did not, then there would be an infinit loop
20
21 positiveInteger--
22 if (positiveInteger + 1 == 5) {
23 continue
24 }
25 console.log(`Current variable value: ${positiveInteger + 1}`)
26}
27
28console.log("\nDone.")
1#!/usr/bin/env python3
2"""
3Created by: Mr. Coxall
4Created on: Sep 2020
5This module uses a continue statement
6"""
7
8
9def main() -> None:
10 """The main() function uses a continue statement, returns None."""
11
12 # input
13 positive_integer = int(input("Enter a count-down number (ex: 10): "))
14 print("")
15
16 # process & output
17 while positive_integer >= 0:
18 # yes, this is the exception on placing the counter at the top
19 # if you did not, then there would be an infinit loop
20 positive_integer -= 1
21 if positive_integer + 1 == 5:
22 continue
23 print(f"Current variable value: {positive_integer + 1}")
24
25 print("\nDone.")
26
27
28if __name__ == "__main__":
29 main()
Example Output
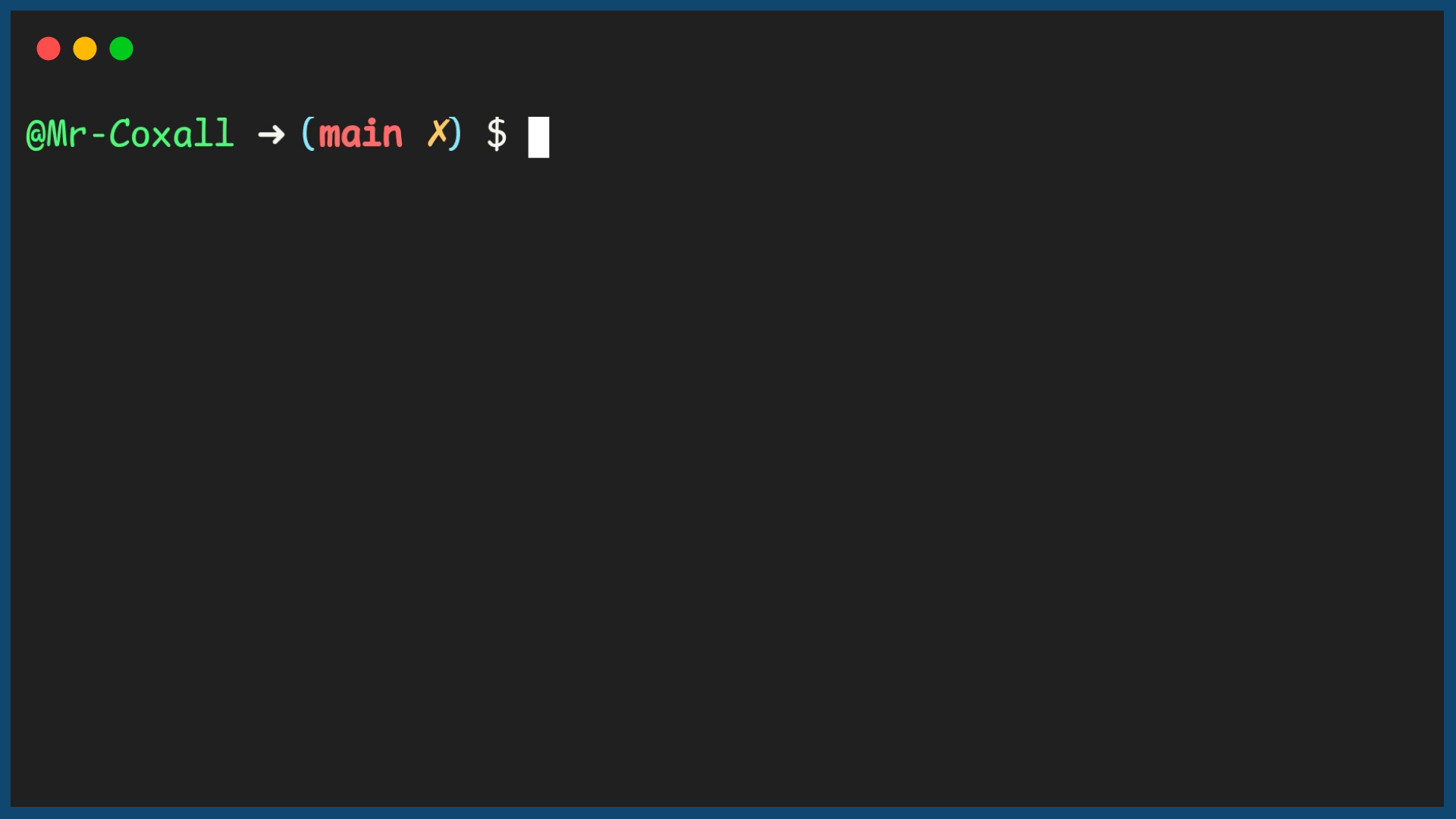