For Loop
The For loop is another repetition structure. The advantage of using the For loop is that the structure itself keeps track of incrimenting the counter, so you do not have to. Usually by default, the counter is just incrimented by 1 each time you go through the loop. As normal, there is some way to exit the loop, usually by some kind of Boolean expression. Some For loops allow you to just specify how many times you would like to do the loop, by providing a number and no Boolean expression.
To use the For…Next loop, there will also be a loop counter that keeps track of how many times through the loop the program has executed. Each time the code inside the loop is executed, the loop counter is automatically incremented by 1. Then an expression checks to see that the predetermined number of times has been reached.
The For loop (in most computer programming languages) takes the generic form of:
or
In this example program, the user is asked to enter a posative integer and the program will count how many times it goes through the loop until it reaches that number.
Top-Down Design for the For loop
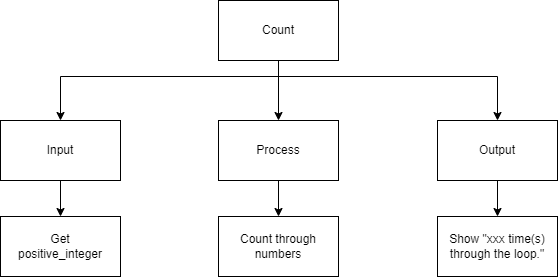
Flowchart for the For loop
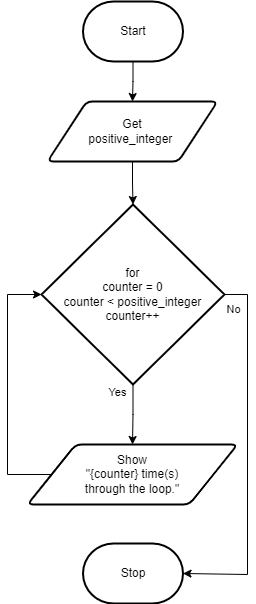
Pseudocode for the For loop
Code for the For loop
1// Copyright (c) 2020 Mr. Coxall All rights reserved.
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program uses a for loop
6
7#include <stdio.h>
8
9int main() {
10 // this function uses a for loop
11 int positiveInteger;
12
13 // input
14 printf("Enter how many times to repeat: ");
15 scanf("%d", &positiveInteger);
16 printf("\n");
17
18 // process & output
19 for (int loopCounter = 0; loopCounter < positiveInteger; loopCounter++) {
20 printf("%d time(s) through the loop.\n", loopCounter);
21 }
22
23 printf("\nDone.\n");
24 return 0;
25}
1// Copyright (c) 2020 St. Mother Teresa HS All rights reserved.
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program uses a for loop
6
7#include <iostream>
8
9int main() {
10 // this function uses a for loop
11 int positiveInteger;
12
13 // input
14 std::cout << "Enter how many times to repeat: ";
15 std::cin >> positiveInteger;
16 std::cout << std::endl;
17
18 // process & output
19 for (int loopCounter = 0; loopCounter < positiveInteger; loopCounter++) {
20 std::cout << loopCounter <<" time through loop." << std::endl;
21 }
22
23 std::cout << "\nDone." << std::endl;
24 return 0;
25}
1/* Created by: Mr. Coxall
2 * Created on: Sep 2020
3 * This program uses a for loop
4*/
5
6using System;
7
8/*
9 * The Program class
10*/
11class Program {
12 static void Main() {
13 // this function uses a do ... while loop
14
15 int positiveInteger;
16
17 // input
18 Console.Write("Enter how many times to repeat: ");
19 positiveInteger = Convert.ToInt32(Console.ReadLine());
20 Console.WriteLine();
21
22 // process & output
23 for (int loopCounter = 0; loopCounter < positiveInteger; loopCounter++) {
24 Console.WriteLine(loopCounter + " time(s) through the loop.");
25 }
26
27 Console.WriteLine("\nDone.");
28 }
29}
1/**
2 * Created by: Mr. Coxall
3 * Created on: Sep 2020
4 * This program uses a for loop
5 */
6
7package main
8
9import (
10 "fmt"
11)
12
13func main() {
14 // this function uses a for loop
15
16 var counter int // in go no need to set to 0, it automaticall is!
17 var positiveInteger int
18
19 // input
20 fmt.Print("Enter how many times to repeat: ")
21 fmt.Scan(&positiveInteger)
22 fmt.Println()
23
24 // process & output
25 for counter < positiveInteger {
26 fmt.Printf("%d time(s) through the loop.\n", counter)
27 counter++ // a short form for: counter = counter + 1
28 }
29
30 fmt.Println("\nDone.")
31}
1/*
2 * This program uses a for loop
3 *
4 * @author Mr Coxall
5 * @version 1.0
6 * @since 2020-09-01
7 */
8
9import java.util.Scanner;
10
11public class Main {
12 public static void main(String[] args) {
13 // this function uses a for loop
14
15 // create Scanner object for user input
16 Scanner scanner = new Scanner(System.in);
17
18 // input
19 System.out.print("Enter how many times to repeat: ");
20 String positiveIntegerStr = scanner.nextLine();
21 System.out.println();
22
23 // process & output
24 int positiveInteger = Integer.parseInt(positiveIntegerStr);
25
26 for (int loopCounter = 0; loopCounter < positiveInteger; loopCounter++) {
27 System.out.println("%d time(s) through the loop.".formatted(loopCounter));
28 }
29
30 // close the Scanner object
31 scanner.close();
32 System.out.println("\nDone.");
33 }
34}
1/* Created by: Mr. Coxall
2 * Created on: Sep 2020
3 * This program uses a for loop
4 */
5
6const prompt = require("prompt-sync")()
7
8let counter = 0
9
10// input
11const positiveIntegerStr = prompt("Enter how many times to repeat: ")
12console.log("")
13
14// process & output
15const positiveInteger = parseInt(positiveIntegerStr)
16
17for (var loopCounter = 0; loopCounter < positiveInteger; loopCounter++) {
18 console.log(`${loopCounter} time(s) through the loop.`)
19}
20
21console.log("\nDone.")
1#!/usr/bin/env python3
2"""
3Created by: Mr. Coxall
4Created on: Sep 2020
5This module uses a for loop
6"""
7
8
9def main() -> None:
10 """The main() function uses a for loop, returns None."""
11
12 # input
13 positive_integer = int(input("Enter how many times to repeat: "))
14 print("")
15
16 # process & output
17 for loop_counter in range(positive_integer):
18 print(f"{loop_counter} time through loop.")
19
20 print("\nDone.")
21
22
23if __name__ == "__main__":
24 main()
Example Output
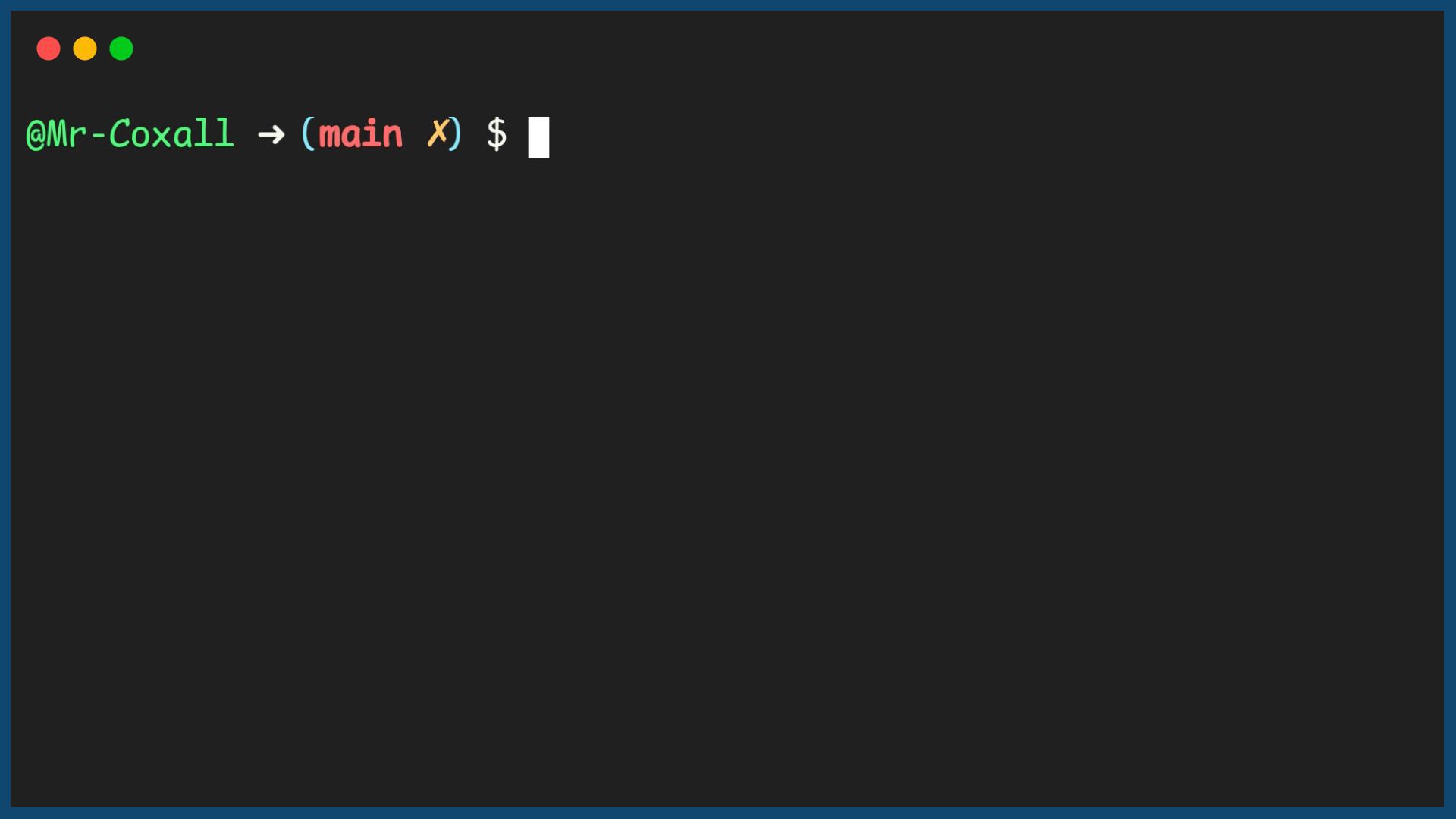