Nested If Statements
Sometimes a single if statement, even a long If…Then…ElseIf…ElseIf…Else is not a suitable structure to model your problem. Sometimes after one decision is made, there is another second decision that must follow. In these cases, if statements can be nested within if statements (or other structures as we will see later).
The nested if statements (in most computer programming languages), takes the generic form of:
IF (Boolean expression A) THEN
statement(s)
IF (Boolean expression B) THEN
statement(s)
ELSE
Alternate statements to be performed
ENDIF
ELSE
Alternate statements to be performed
ENDIF
In this example problem a school is going to sell cookies to raise money. If a student sells 20 or more boxes, they get a prize. If they sell less than 30, they get a, “small” prize. If they sell more than 30, they get a, “large” prize. (Yes you could use an If…Then…ElseIf… statement.)
Top-Down Design for Nested If statement
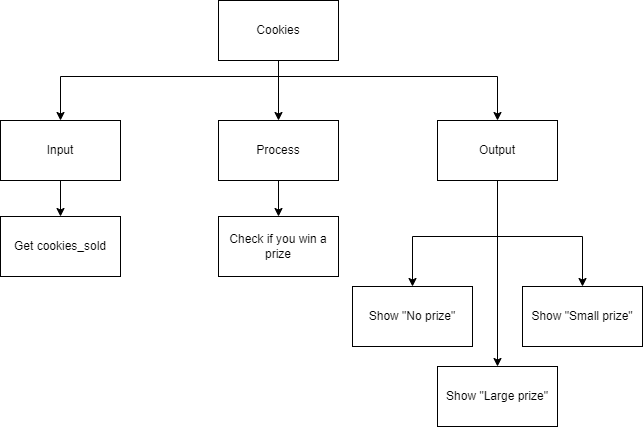
Flowchart for Nested If statement
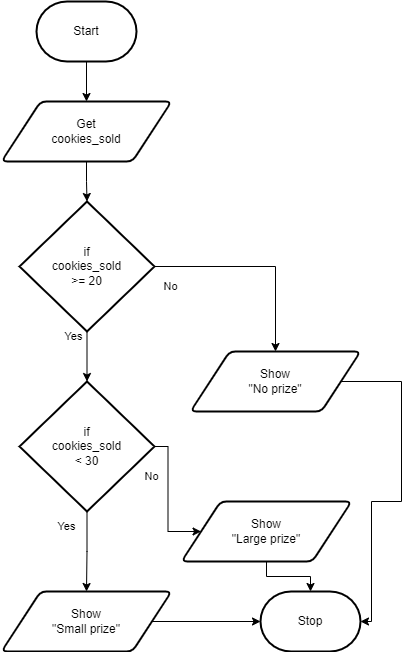
Pseudocode for Nested If statement
GET cookies_sold
IF (cookies_sold >= 20) THEN
IF (cookies_sold < 30) THEN
SHOW “Small prize!”
ELSE
SHOW “Large prize!”
ENDIF
ELSE
SHOW “No prize.”
ENDIF
Code for Nested If statement
1// Copyright (c) 2020 Mr. Coxall All rights reserved.
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program uses a nested if statement
6
7#include <stdio.h>
8
9int main() {
10 // this function uses a nested if statement
11 int cookiesSold;
12
13 // input
14 printf("Enter the number of boxes of cookies you sold: ");
15 scanf("%d", &cookiesSold);
16 printf("\n");
17
18 // process & output
19 if (cookiesSold >= 20) {
20 if (cookiesSold < 30) {
21 printf("You get a small prize.\n");
22 } else {
23 printf("You get a large prize.\n");
24 }
25 } else {
26 printf("No prize.\n");
27 }
28
29 printf("\nDone.\n");
30 return 0;
31}
1// Copyright (c) 2020 St. Mother Teresa HS All rights reserved.
2//
3// Created by: Mr. Coxall
4// Created on: Sep 2020
5// This program uses a nested if statement
6
7#include <iostream>
8
9int main() {
10 // this function uses a nested if statement
11 int cookiesSold;
12
13 // input
14 std::cout << "Enter the number of boxes of cookies you sold: ";
15 std::cin >> cookiesSold;
16 std::cout << std::endl;
17
18 // process & output
19 if (cookiesSold >= 20) {
20 if (cookiesSold < 30) {
21 std::cout << "You get a small prize." << std::endl;
22 } else {
23 std::cout << "You get a large prize." << std::endl;
24 }
25 } else {
26 std::cout << "No prize." << std::endl;
27 }
28
29 std::cout << "\nDone." << std::endl;
30 return 0;
31}
1/* Created by: Mr. Coxall
2 * Created on: Sep 2020
3 * This program uses a nested if statement
4*/
5
6using System;
7
8/*
9 * The Program class
10*/
11class Program {
12 static void Main() {
13 // this function uses a nested if statement
14
15 int cookiesSold;
16
17 // input
18 Console.Write("Enter the number of boxes of cookies you sold: ");
19 cookiesSold = Convert.ToInt32(Console.ReadLine());
20 Console.WriteLine();
21
22 // process & output
23 if (cookiesSold >= 20) {
24 if (cookiesSold < 30) {
25 Console.WriteLine("You get a small prize.");
26 } else {
27 Console.WriteLine("You get a large prize.");
28 }
29 } else {
30 Console.WriteLine("No prize.");
31 }
32
33 Console.WriteLine("\nDone.");
34 }
35}
1/**
2 * Created by: Mr. Coxall
3 * Created on: Sep 2020
4 * This program uses a nested if statement
5 */
6
7package main
8
9import (
10 "fmt"
11)
12
13func main() {
14 // this function uses a nested if statement
15
16 var cookiesSold int
17
18 // input
19 fmt.Print("Enter the number of boxes of cookies you sold: ")
20 fmt.Scan(&cookiesSold)
21 fmt.Println()
22
23 // process & output
24 if cookiesSold >= 20 {
25 if cookiesSold < 30 {
26 fmt.Println("You get a small prize.")
27 } else {
28 fmt.Println("You get a large prize.")
29 }
30 } else {
31 fmt.Println("No prize.")
32 }
33
34 fmt.Println("\nDone.")
35}
1/*
2 * This program uses a nested if statement
3 *
4 * @author Mr Coxall
5 * @version 1.0
6 * @since 2020-09-01
7 */
8
9import java.util.Scanner;
10
11public class Main {
12 public static void main(String[] args) {
13 // this function uses a nested if statement
14
15 // create Scanner object for user input
16 Scanner scanner = new Scanner(System.in);
17
18 // input
19 System.out.print("Enter the number of boxes of cookies you sold: ");
20 String cookiesSoldStr = scanner.nextLine();
21 System.out.println();
22
23 // process & output
24 int cookiesSold = Integer.parseInt(cookiesSoldStr);
25
26 if (cookiesSold >= 20) {
27 if (cookiesSold < 30) {
28 System.out.println("You get a small prize.");
29 } else {
30 System.out.println("You get a large prize.");
31 }
32 } else {
33 System.out.println("No prize.");
34 }
35
36 // close the Scanner object
37 scanner.close();
38 System.out.println("\nDone.");
39 }
40}
1/* Created by: Mr. Coxall
2 * Created on: Sep 2020
3 * This program uses a nested if statement
4 */
5
6const prompt = require("prompt-sync")()
7
8// input
9const cookiesSoldStr = prompt("Enter the number of boxes of cookies you sold: ")
10console.log("")
11
12// process & output
13const cookiesSold = parseInt(cookiesSoldStr)
14
15if (cookiesSold >= 20) {
16 if (cookiesSold < 30) {
17 console.log("You get a small prize.")
18 } else {
19 console.log("You get a large prize.")
20 }
21} else {
22 console.log("No prize.")
23}
24
25console.log("\nDone.")
1#!/usr/bin/env python3
2"""
3Created by: Mr. Coxall
4Created on: Sep 2020
5This module uses a nested if statement
6"""
7
8
9def main() -> None:
10 """The main() function uses a nested if statement, returns None."""
11
12 # input
13 cookies_sold = int(input("Enter the number of boxes of cookies you sold: "))
14 print("")
15
16 # process & output
17 if cookies_sold >= 20:
18 if cookies_sold < 30:
19 print("You get a small prize.")
20 else:
21 print("You get a large prize.")
22 else:
23 print("No prize.")
24
25 print("\nDone.")
26
27
28if __name__ == "__main__":
29 main()
Example Output
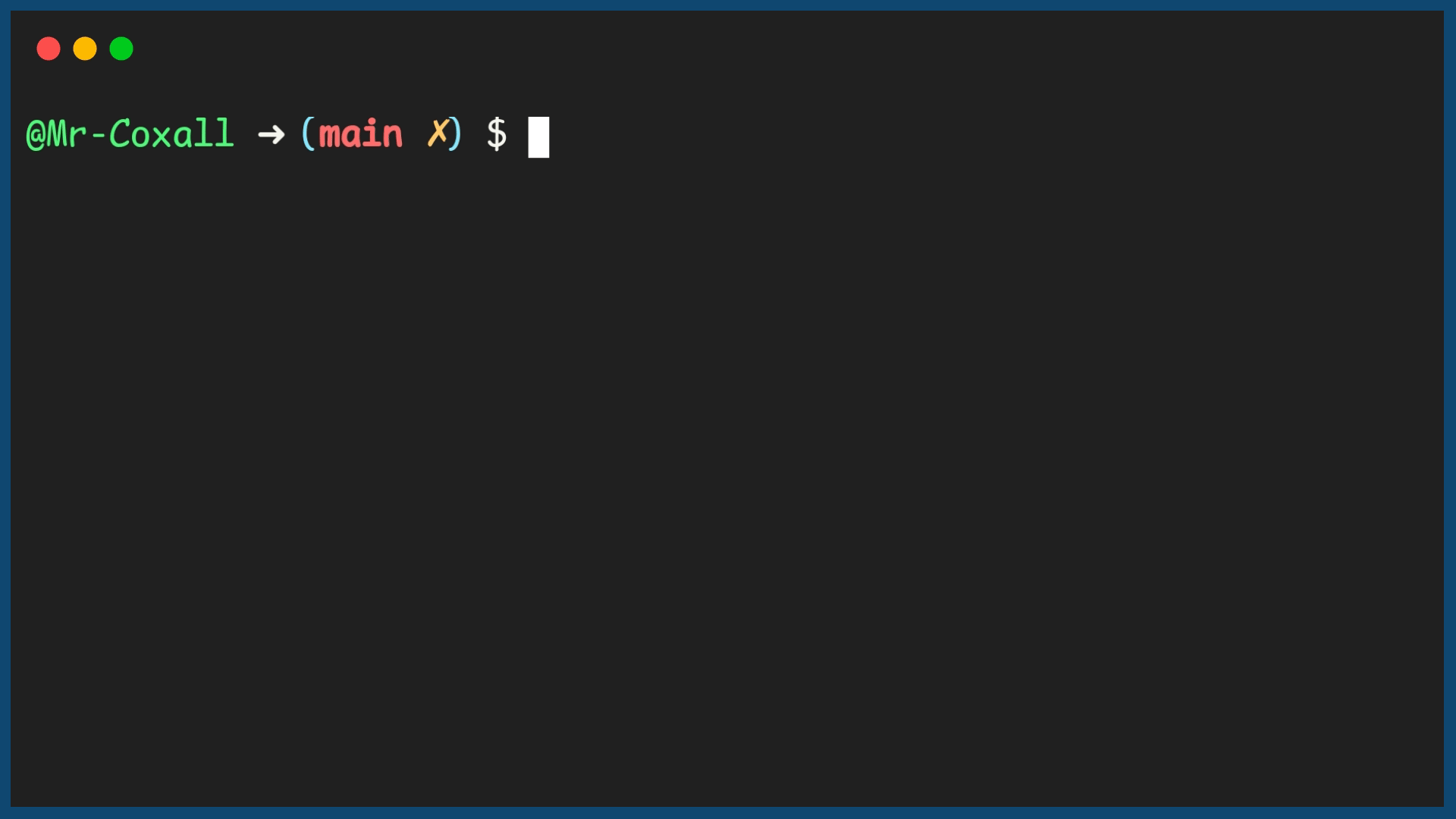